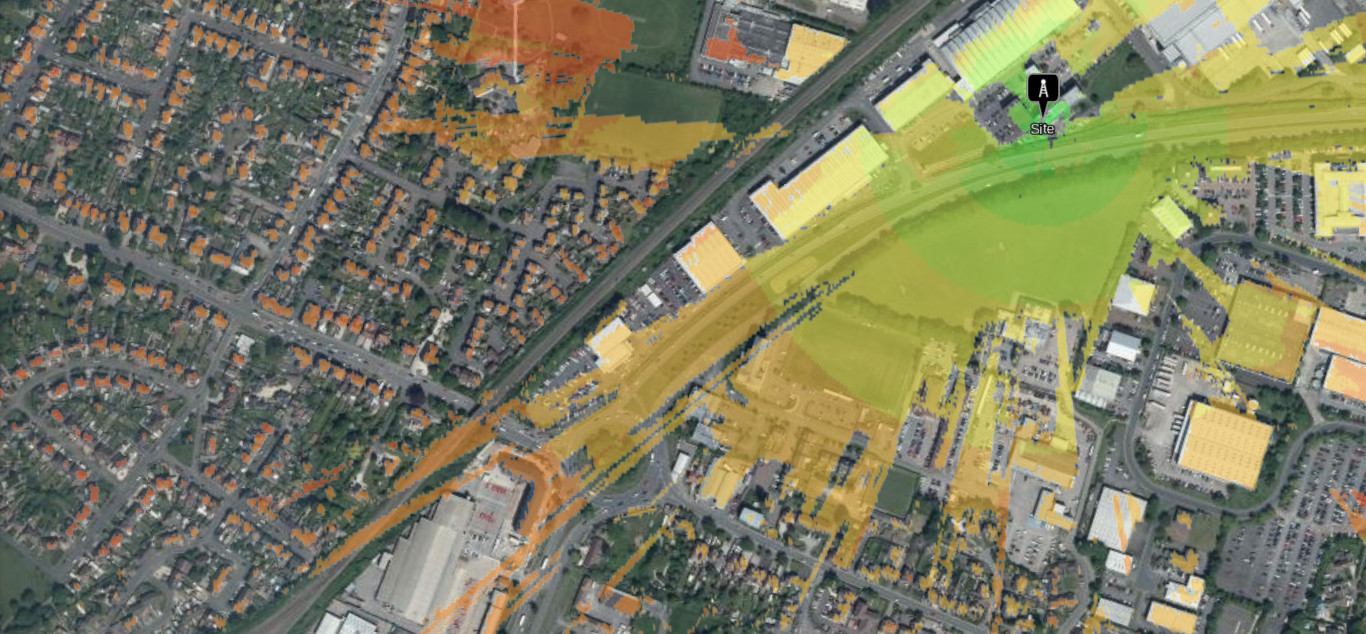
Our fast GPU engine is perfect for modelling wireless coverage
We have developed the next generation of fast radio simulation engines for urban modelling with NVIDIA CUDA technology and Graphics Processing Units (GPUs).
The engine was made to meet demand across many sectors, especially FWA, 5G and CBRS for speed and accuracy.
As well as fast viewsheds, it enables a new automated best-site-analysis capability, which will accelerate site selection and improve efficiency whilst keeping a human in the loop. As we can do clutter attenuation, it’s suitable for VHF and LPWAN also.
Designed for 5G
5G networks are much denser than legacy standards due to the limited range of mmWave signals, necessary for high bandwidth data. The same limitation means these signals are very sensitive to obstructions, and Line of Sight (LOS) coverage is essential for performance.
With 1 metre accuracy and support for LiDAR, 3D clutter and custom clutter profiles, you can model rural and urban areas with high precision.
We can do Trees too
Unlike simplistic viewshed GPU tools designed for speed, we can model actual tree attenuation for beyond line of sight sub-GHz signals such as LPWAN and VHF. Trees can be configured as clutter profiles, along with shrubs, swamps, urban areas and 18 classes of Land cover and custom clutter.
Area coverage
The simplest mode is a fast “2.5D” viewshed (with a path loss model) which creates a point-to-multipoint heatmap around a given site using LiDAR data. Ours has better Physics than some of the “line of sight” eye candy on the market and doesn’t have trouble with Sub-GHz frequencies which are harder to model accurately.
This is up to 50 times faster than our multi-threaded CPU engine, SLEIPNIR.
In this mode we can do diffraction and material attenuation with our custom clutter classes.
Best site analysis
Best Site Analysis (BSA) is a monte-carlo analysis technique across a wide area of interest to identify the best locations for a transmitter. This can be done quickly with a new /bsa API call. The output will identify optimal sites, and just as important, inefficient sites.
This feature is powerful for IoT gateway placement, 5G deployments and ad-hoc networking where the best site might presently be determined by a map study based on contours as opposed to a LiDAR model.
High speed
Our GPU engine is up to 50 times faster through the API than the current (CPU) engine SLEIPNIRTM
By harnessing the power of high performance graphics cards, we are able to complete high resolution LiDAR plots in near real time, negating the need for a “go” button, or even a progress bar. This speed enables API integration with vehicles and robots which will need to model wireless propagation quickly to make better decisions, especially when they’re off the grid. It was designed around consumer grade cards like the GeForce series but supports enterprise Tesla grade cards due to our card agnostic design.
Economical
Our implementation is efficient by design. We want speed to model wireless coverage but not if it requires kilowatts of power. During testing we worked with older GeForce consumer cards and were able to model millions of points in several milliseconds with less than 50W of power. Or in other words, the same power as flicking a light bulb on and off.
Any fool can buy large cards and waste electricity, but we’re proud to have a solution which is fast and economical. This also means it can be run on a laptop as it’s available now as our SOOTHSAYER product.
Open API
The GPU engine is an “engine” parameter in our /area API so you can use it from any interface (or your own custom interface) by setting the engine option in the request body. The OpenAPI 3.0 compliant API returns JSON which contains a PNG image so for existing API integrations using our PNG layers there will be no code changes required to enable it.
Self-hosted GPU server
Instead of buying milk every month you can buy the cow. We also sell SOOTHSAYER which is a self-hosted server with our GPU engine onboard. It supports NVidia GPU cards from Maxwell architecture onwards and most enterprise Hypervisors like ESXi and Proxmox. You get to use your existing LiDAR data too, so you’re not buying it twice.
To see how easy it is to setup a GPU card with SOOTHSAYER we’ve made a video:
Accessible
Using GPU cards to model Physics, including EM propagation, is an established concept dating back 20 years, despite businessmen claiming otherwise. What is novel here, is making this exciting technology accessible to users priced out of premium tools.
Staying true to CloudRF’s accessible and affordable principles, we’ve included it in our service as an optional processing engine.