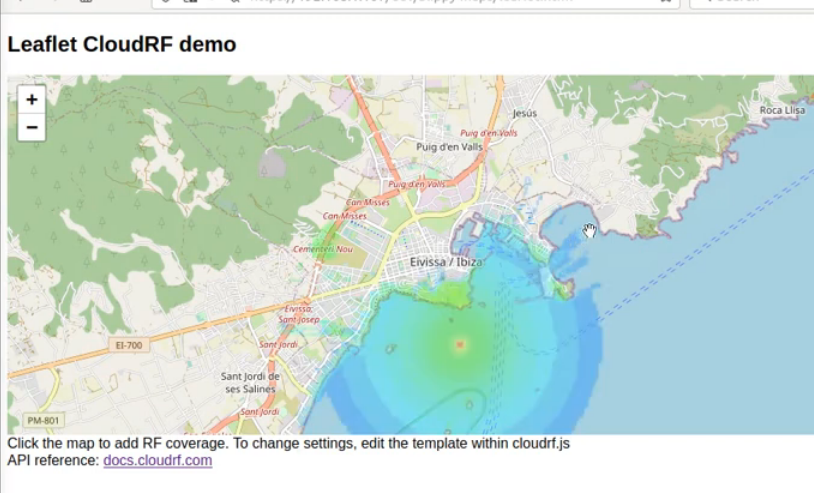
LeafletJS (32k stars on Github) and it’s predecessor Openlayers (8k stars) are very popular web mapping libraries in use with companies and organisations the world over. They provide powerful, fast and easy client side 2D maps written in Javascript which can show almost any type of data…
Whilst typically used on public facing web maps with public tile servers like OpenStreetMap, the libraries are versatile enough to be used on private networks with private WMS or TMS tile servers.
Adding the CloudRF API is made possible through our open standards such as JSON, PNG, EPSG:3857, EPSG:4326 and HTTP. In this post we show two new examples for integrating the Area API.
LeafletJS Area API integration
The workflow for Leaflet is as follows:
- Fetch the selected location, in this case a cursor click and transform to EPSG:4326 co-ordinates (Latitude, Longitude)
function onMapClick(e) {
addRFLayer(e.latlng.lat,e.latlng.lng);
}
map.on('click', onMapClick);
- Take a pre-built JSON object containing a complete request representing “a radio”. Replace the transmitter lat and lon fields with our latitude and longitude.
- Send the full request as a HTTP POST along with an API key in the header to https://api.cloudrf.com/area
var xhr = new XMLHttpRequest();
xhr.withCredentials = false;
xhr.addEventListener("readystatechange", function() {
if(this.readyState === 4) {
AddLayerToMap(this.responseText);
}
});
xhr.open("POST", "https://api.cloudrf.com/area");
xhr.setRequestHeader("key", key);
xhr.send(request);
- When the response returns, parse the JSON to extract the PNG_Mercator URL and bounds array.
- Using Leaflet’s imageOverlay method, place the image upon the map, tweaking the opacity to 50% in the process, with the bounds rearranged in [SOUTH, WEST], [NORTH, WEST] order.
var bounds = json.bounds; // CloudRF uses NORTH,EAST,SOUTH,WEST
var imageBounds = [[bounds[2], bounds[3]], [bounds[0], bounds[1]]];
L.imageOverlay(json.PNG_Mercator, imageBounds).setOpacity(0.5).addTo(map);
Openlayers Area API integration
The workflow for Openlayers is as follows:
- Fetch the selected location, in this case a cursor click and transform to EPSG:4326 co-ordinates (Latitude, Longitude)
map.on('click', function(evt){
var pt = ol.proj.transform(evt.coordinate, 'EPSG:3857', 'EPSG:4326');
addRFLayer(pt[1],pt[0]);
});
var xhr = new XMLHttpRequest();
xhr.withCredentials = false;
xhr.addEventListener("readystatechange", function() {
if(this.readyState === 4) {
AddLayerToMap(this.responseText);
}
});
xhr.open("POST", "https://api.cloudrf.com/area");
xhr.setRequestHeader("key", key);
xhr.send(request);
var imageLayer = new ol.layer.Image({
opacity: 0.5,
source: new ol.source.ImageStatic({
url: json.PNG_Mercator,
projection: map.getView().getProjection(),
imageExtent: ol.proj.transformExtent(boundsWSEN, 'EPSG:4326', 'EPSG:3857')
})
});
map.addLayer(imageLayer);
Source code
Get examples for both slippy maps at https://github.com/Cloud-RF/CloudRF-API-clients
API reference
API documentation and examples are at https://docs.cloudrf.com
A Swagger OpenAPI3.0 specification is at https://cloudrf.com/documentation/developer/swagger-ui/
Other uses
Beyond heatmaps here are a few suggestions:
- Draw a polyline to simulate a link with the /path API
- Draw a freehand route to test a route with the /points API
- Draw a sectorial antenna as a polygon with an azimuth and beamwidth and send to the /area API
- Use the Trilateration colour schema to model a detected signal or a distant handset with the /area API